How Comment GraphQL API works
Author:
Kirill Gaiduk
Changed on:
9 Dec 2024
Overview
The Comment GraphQL API enables you to Create, Update, and Query comments linked to various entities within the Fluent platform.
Prerequisites
- You should have knowledge of the GraphQL API, including:
- You should have knowledge of Users, Roles, and Permissions
Comment GraphQL API Overview
Authors:
Girish Padmanabha, Kirill Gaiduk
Changed on:
20 Feb 2025
Overview
The Comment GraphQL API allows customers to manage Comments across various Entities.
Multiple Comments can be added against the same Entity, with each Comment automatically capturing:
- The timestamp
- The User who created it (Permission is required to access the User data)
`USER_VIEW`
This feature allows Users to record specific business actions and events, providing a comprehensive history of interactions and updates.
Key points
- Add Comments to any Entity Type, enabling detailed documentation of business actions
- Specific Permissions are required for Comment operations
- Use the and
`createComment`
Mutations to manage Comments`updateComment`
- Use the and
`commentById`
Queries to retrieve Comments`comments`
What are Comments for?
A Comment provides an additional piece of information to the Entity it is related to.
When creating a Comment, you can associate it with:
- An Entity Type
- Entity Id or Entity Reference
Making it retrievable via the `comments`
Permissions
The following Permissions are required for different operations on Comments:
`COMMENT_CREATE`
`COMMENT_UPDATE`
`COMMENT_VIEW`
`USER_VIEW`
Features
The Comment GraphQL API enables the following functionality:
- Create Comment
TheMutation allows the creation of a Comment against a known Entity.`createComment`
- Update Comment
TheMutation allows for the updating of an existing Comment object.`updateComment`
- Get Comment by Id
TheQuery retrieves a Comment based on a provided Id.`commentById`
- Query Comments
TheQuery retrieves Comments based on various filter criteria.`comments`
Reference Usage
Here is a collection of common scenarios for the Comment GraphQL API usage:
- Order Entity:
- Customer service representatives can add Comments regarding customer interactions or actions taken on an Order
- Comments can document the business status updates or any issues related to the Order processing
- Fulfillment Entity:
- Store Users can add Comments when fulfilling Orders, noting any special instructions or issues encountered during the fulfillment process
- Credit Memo Entity:
- Financial teams can document reasons for issuing Credit Memos and any follow-up actions required
- Return Order Entity:
- Comments can be added detailing the reasons for Returns and the steps taken to process them
- Location Entity:
- Store managers can add Comments about:
- Inventory status
- Store operations
- Or any Location-specific updates
- Store managers can add Comments about:
This flexibility allows the capture of essential information at various stages of business processes, ensuring that all relevant data is saved and can be retrieved when needed.
Related content
Create Comment
Author:
Kirill Gaiduk
Changed on:
20 Feb 2025
Overview
The `createComment`
Prerequisites
Specific Permissions are required for creating Comments:
`COMMENT_CREATE`
`COMMENT_VIEW`
Key points
- Use the Mutation to create a Comment against a known Entity
`createComment`
- Manage the Comment Permissions at the Account or Retailer level
- Apply the "Retailer-specific Comment Permission Check" validation logic with the Setting (
`fc.graphql.comment.access`
value)`retailer`
- Created Comments inherit the of the associated Entity
`retailerId`
Inputs
The Input fields for creating a Comment are defined with the `createCommentInput`
Field | Type | Description | Notes |
| String! | Type of the Entity | For example:
Max character limit: 255 |
| ID | Id of the Entity | While the type of this field is ID, it currently only supports Integer values |
| String | Reference of the Entity | Max character limit: 255 |
| String! | Comment text | Max character limit: 200 |
Validation
Comment Permissions could be managed at the Account or Retailer level, which is controlled via the `fc.graphql.comment.access`
The
`retailer`
`createComment`
1. Determine the `retailerId`
of the Entity (associated with the Comment)
`retailerId`
Entity Type Validation
- The given (Input) is compared with the existing Types (from the GraphQL Schema):
`entityType`
- Case is ignored (e.g., matches
`ORDER`
)`Order`
- Underscore is ignored (e.g., matches
`CREDIT_MEMO`
)`CreditMemo`
- Known exception: will be recognized as
`FULFILMENT_OPTIONS`
Type`FulfilmentOption`
- Case is ignored (e.g.,
- The corresponding Database Entity Class is determined for the found GraphQL Type (e.g., Type corresponds to the
`ORDER`
Class)`CustomerOrderEntity`
Entity Id or Reference Validation
- The Create Comment Payload could contain:
- only
`entityId`
- only
`entityRef`
- Both and
`entityId`
`entityRef`
- is always considered first (to define the corresponding Entity for Comment creation)
`entityId`
- is considered when
`entityRef`
was not provided`entityId`
Entity Retailer Id Validation
- The value of the Entity found with the provided
`retailerId`
,`entityType`
/`entityId`
is automatically retrieved:`entityRef`
- For Entity Types with the direct Retailer association, e.g., is taken from the
`retailerId`
field value for Orders`order.retailer`
- For Entity Types without the direct Retailer association, the is attempted to be taken from a "Parent" Entity. For example, find the Fulfillment (with the provided
`retailerId`
,`entityType`
/`entityId`
) --> find its "Parent" Order --> get the`entityRef`
from the Order`retailerId`
- For Entity Types with the direct Retailer association, e.g.,
- Comment field is filled in with the associated Entity Retailer value found.
`retailerId`
- Comment field remains empty when:
`retailerId`
- The associated Entity was not found
`retailerId`
- The associated Entity is a cross-Retailer one (e.g., Inventory Domain Entities, like Inventory Position, Virtual Catalog, etc.)
- The Setting value is set to
`fc.graphql.comment.access`
`account`
- The associated Entity
2. Compare the querying User `retailerId`
to the associated Entity `retailerId`
`retailerId`
`retailerId`
The User
`retailerId`
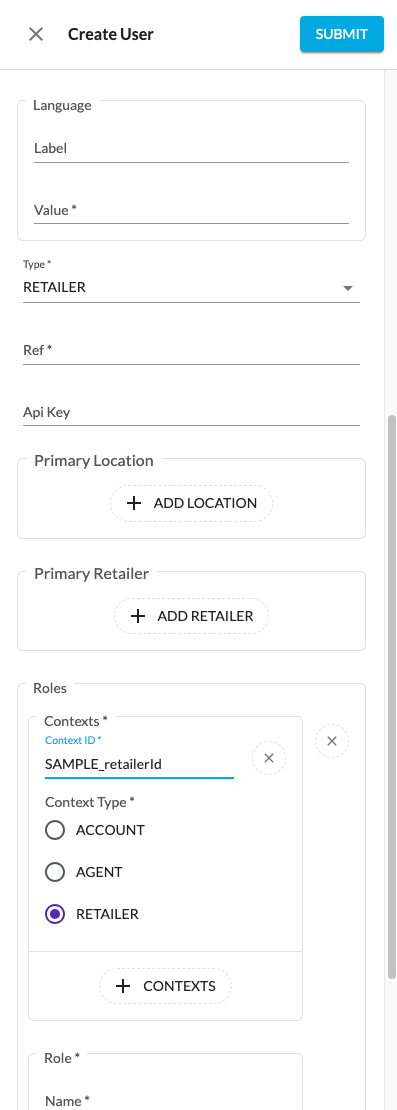
A Comment is created upon the mentioned (User and Entity)
`retailerId`
Response
The response consists of the details of the Comment:
Field | Type | Description | Notes |
| ID! | Id of the Comment | |
| String! | Type of the Entity | For example:
|
| ID | Id of the Entity | |
| String | Reference of the Entity | |
| String! | Comment text | Max character limit: 200 |
| DateTime | Time of the Comment creation | |
| DateTime | Time of the Comment last update | |
| User | The author of the Comment |
|
Sample Payload
1mutation createComment ($input: CreateCommentInput) {
2 createComment (input: $input) {
3 id
4 entityType
5 entityRef
6 entityId
7 text
8 createdOn
9 updatedOn
10 }
11}
Language: graphqlschema
Name: Sample createComment Mutation
Description:
Creating a Comment.
API Endpoint:
`POST: {{fluentApiHost}}/graphql`
1{
2 "input": {
3 "entityType": "ORDER",
4 "entityId": "123",
5 "text": "Sample Comment for the Order"
6 }
7}
Language: graphqlschema
Name: Sample GraphQL Variables for the createComment Mutation
Description:
Creating a Comment for the Order with a specified Id.
1{
2 "data": {
3 "createComment": {
4 "id": "26963",
5 "entityType": "ORDER",
6 "entityRef": null,
7 "entityId": "123",
8 "text": "Sample Comment for the Order",
9 "createdOn": "2024-12-09T09:15:57.396Z",
10 "updatedOn": "2024-12-09T09:15:57.396Z"
11 }
12 }
13}
Language: graphqlschema
Name: createComment Mutation Response Example
Description:
Creating a Comment for the Order with a specified Id.
Update Comment
Author:
Kirill Gaiduk
Changed on:
20 Feb 2025
Overview
The `updateComment`
Prerequisites
Specific Permissions are required for updating Comments:
`COMMENT_UPDATE`
`COMMENT_VIEW`
Key points
- Use the Mutation to update an existing Comment
`updateComment`
- Manage the Comment Permissions at the Account or Retailer level
- Apply the "Retailer-specific Comment Permission Check" validation logic with the Setting (
`fc.graphql.comment.access`
value)`retailer`
Inputs
The Input fields for updating a Comment are defined with the `UpdateCommentInput`
Field | Type | Description | Notes |
| ID! | Id of the Comment | |
| String! | Comment text | Max character limit: 200 |
Validation
Comment Permissions could be managed at the Account or Retailer level, which is controlled via the `fc.graphql.comment.access`
The
`retailer`
`updateComment`
- A target Comment is found by its Id (input)
- A Comment Entity field stores a Retailer of the associated Entity
`retailerId`
- The Comment is compared to the querying User
`retailerId`
(defined with the User Role Context Id)`retailerId`
- The Comment is updated upon the mentioned (User and Comment) 's match
`retailerId`
Response
The response consists of the details of the Comment:
Field | Type | Description | Notes |
| ID! | Id of the Comment | |
| String! | Type of the Entity | For example:
|
| ID | Id of the Entity | |
| String | Reference of the Entity | |
| String! | Comment text | Max character limit: 200 |
| DateTime | Time of the Comment creation | |
| DateTime | Time of the Comment last update | |
| User | The author of the Comment |
|
Sample Payload
1mutation updateComment ($input: UpdateCommentInput) {
2 updateComment (input: $input) {
3 id
4 entityType
5 entityRef
6 entityId
7 text
8 createdOn
9 updatedOn
10 }
11}
Language: graphqlschema
Name: Sample updateComment Mutation
Description:
Updating the Comment.
API Endpoint:
`POST: {{fluentApiHost}}/graphql`
1{
2 "input": {
3 "id": "26963",
4 "text": "Sample Comment"
5 }
6}
Language: graphqlschema
Name: Sample GraphQL Variables for the updateComment Mutation
Description:
Updating the Comment with a specified Id.
1{
2 "data": {
3 "updateComment": {
4 "id": "26963",
5 "entityType": "ORDER",
6 "entityRef": null,
7 "entityId": "123",
8 "text": "Sample Comment",
9 "createdOn": "2024-12-09T09:15:57.396Z",
10 "updatedOn": "2024-12-09T09:22:57.791Z"
11 }
12 }
13}
Language: graphqlschema
Name: updateComment Mutation Response Example
Description:
Updating the Comment with a specified Id.
Get Comment by Id
Author:
Kirill Gaiduk
Changed on:
20 Feb 2025
Overview
The `commentById`
Prerequisites
- Permission is required for retrieving a Comment
`COMMENT_VIEW`
Key points
- Use the Query to retrieve an existing Comment
`commentById`
- Manage the Comment Permissions at the Account or Retailer level
- Apply the "Retailer-specific Comment Permission Check" validation logic with the Setting (
`fc.graphql.comment.access`
value)`retailer`
Inputs
The Input arguments for retrieving a single Comment:
Argument | Type | Description |
| ID! | Id of the Comment |
Validation
Comment Permissions could be managed at the Account or Retailer level, which is controlled via the `fc.graphql.comment.access`
The
`retailer`
`commentById`
- A target Comment is found by its Id (input)
- A Comment Entity field stores a Retailer of the associated Entity
`retailerId`
- The Comment is compared to the querying User
`retailerId`
(defined with the User Role Context Id)`retailerId`
- The Comment is retrieved upon the mentioned (User and Comment) 's match
`retailerId`
Response
The response consists of the details of the Comment:
Field | Type | Description | Notes |
| ID! | Id of the Comment | |
| String! | Type of the Entity | For example:
|
| ID | Id of the Entity | |
| String | Reference of the Entity | |
| String! | Comment text | Max character limit: 200 |
| DateTime | Time of the Comment creation | |
| DateTime | Time of the Comment last update | |
| User | The author of the Comment |
|
Sample Payload
1query commentById ($id: ID!) {
2 commentById (id: $id) {
3 id
4 entityType
5 entityId
6 entityRef
7 text
8 createdOn
9 updatedOn
10 user {
11 username
12 id
13 ref
14 primaryEmail
15 firstName
16 lastName
17 timezone
18 }
19 }
20}
Language: graphqlschema
Name: Sample commentById Query
Description:
Getting the Comment.
API Endpoint:
`POST: {{fluentApiHost}}/graphql`
1{
2 "id": 123
3}
Language: graphqlschema
Name: Sample GraphQL Variables for the commentById Query
Description:
Getting the Comment with a specified Id.
Query Comments
Author:
Kirill Gaiduk
Changed on:
20 Feb 2025
Overview
The `comments`
Prerequisites
- Permission is required for retrieving Comments
`COMMENT_VIEW`
Key points
- Use the Query to retrieve existing Comments
`comments`
- Manage the Comment Permissions at the Account or Retailer level
- Apply the "Retailer-specific Comment Permission Check" validation logic with the Setting (
`fc.graphql.comment.access`
value)`retailer`
Inputs
The Input arguments for retrieving Comments:
Argument | Type | Description | Notes |
| [String!] | Type of the Entity | For example:
|
| [ID] | Id of the Entity | |
| [String] | Reference of the Entity | |
| [String!] | Comment text | |
| DateRange | Date range for Comments creation | |
| DateRange | Date range for Comments last update | |
| Int | Returns the first n elements from the list | |
| Int | Returns the last n elements from the list | |
| String | Returns the elements in the list that come before the specified global Id | This is a cursor (the value is used for pagination) |
| String | Returns the elements in the list that come after the specified global Id | This is a cursor (the value is used for pagination) |
Validation
Comment Permissions could be managed at the Account or Retailer level, which is controlled via the `fc.graphql.comment.access`
The
`retailer`
`comments`
- Comments are searched by the given Input Arguments
- A Comment Entity field stores a Retailer of the associated Entity
`retailerId`
- For all the found Comments, the Comment is compared to the querying User
`retailerId`
(defined with the User Role Context Id)`retailerId`
- The list of the retrieved Comments contains:
- The Comments for which the mentioned (User and Comment) -s matches
`retailerId`
- The Comments with empty
`retailerId`
- The Comments for which the mentioned (User and Comment)
Response
The response consists of the details of the Comment:
Field | Type | Description | Notes |
| ID! | Id of the Comment | |
| String! | Type of the Entity | For example:
|
| ID | Id of the Entity | |
| String | Reference of the Entity | |
| String! | Comment text | Max character limit: 200 |
| DateTime | Time of the Comment creation | |
| DateTime | Time of the Comment last update | |
| User | The author of the Comment |
|
Sample Payload
1query comments($createdOn: DateRange) {
2 comments(createdOn: $createdOn) {
3 edges {
4 node {
5 id
6 entityId
7 entityRef
8 entityType
9 text
10 createdOn
11 updatedOn
12 }
13 cursor
14 }
15 }
16}
Language: graphqlschema
Name: Sample comments Query
Description:
Getting the Comments created within the specified date range.
API Endpoint:
`POST: {{fluentApiHost}}/graphql`
1{
2 "createdOn": {
3 "from": "2024-11-16T00:32:28.935Z",
4 "to": "2024-11-16T23:40:28.935Z"
5 }
6}
Language: graphqlschema
Name: Sample GraphQL Variables for the comments Query
Description:
Getting the Comments created within the specified date range.