Create Order Wizard
Author:
Siarhei Vaitsiakhovich
Changed on:
7 Feb 2025
Key Points
- The 'Create Order' wizard streamlines the order creation process by guiding users through each step, reducing the chances of errors, and ensuring all necessary information is captured.
- It provides a user-friendly interface that simplifies complex order configurations, making it accessible for users with varying levels of technical expertise.
- The wizard includes validation checks at each step, ensuring that all entered data is accurate and complete before proceeding to the next step.
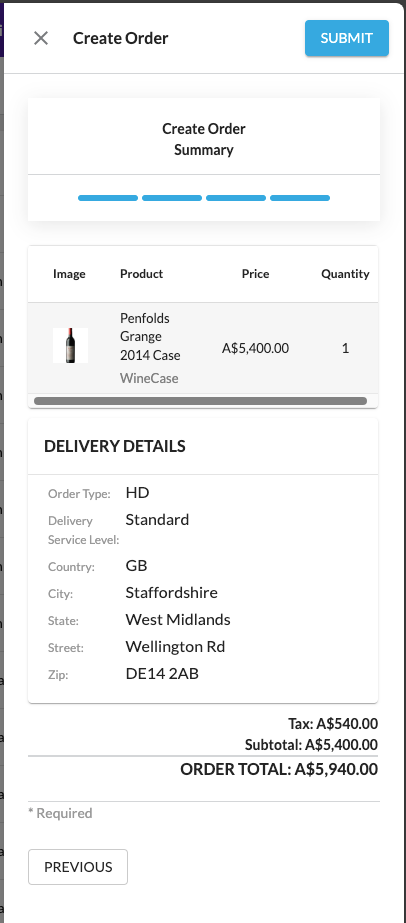
Prerequisites
Steps
Solutions Approach
The Create Order wizard how allows to collect all order-related information before starting order processing. List of collectible information:
- List of Order Items
- Customer Information
- Order Type
- Delivery Information (Customer, Delivery address, or Pickup Location information)
- Information about fulfillment choices (for Mixed Order)
- List of Financial Transactions related to order
Create Order wizard component has to be designed to be integrated into any place of application as user action.
Technical Design Overview
To support the business solution design, the following technical areas need to be enhanced:
- A new customer UI component (by using Component SDK) -
`createOrderWizard`
- Modify application manifest to display new action as user action
- Add a list of settings that are needed for the correct working of the Create Order wizard
- Update of the Language Setting
Each of these tasks will be described below in steps.
UI Component: createOrderWizard
Create Order Wizard component was designed and registered as FieldComponent to be used in mutation user action. Additionally,
`currency`
`currencySymbol`
1// index.tsx
2
3import { FieldRegistry } from 'mystique/registry/FieldRegistry';
4
5import { CreateOrder as CreateOrderField } from './forms/fields/order/СreateOrder/CreateOrder';
6import { TemplateRegistry } from 'mystique/registry/TemplateRegistry';
7import { currency, currencySymbol } from './utils/TemplateUtils';
8
9FieldRegistry.register(['createOrderWizard'], CreateOrderField);
10
11// Templates
12TemplateRegistry.register('currency', currency);
13TemplateRegistry.register(['currencySymbol'], currencySymbol);
14
Language: typescript
Name: Example of new component and templates registration
Description:
[Warning: empty required content area]
UI Component: Known Issues
Part of additional data that is needed for correct work of Create Order Component was hardcoded, so code have to be modified according to specific environment.
Known places:
- Hardcoded Virtual Catalogue Reference
1 await getQuery<NetworkIdsResult>(networkIdsQuery, {
2 ref: `VC:BASE:${activeRetailer}`, // TODO virtual catalogue ref (for example, BASE or VC:BASE)
3 }).then((resp) => {
Language: typescript
Name: src/queries/getBaseLocations.tsx:43
Description:
[Warning: empty required content area]- Hardcoded Product Catalogue Reference
1 await getQuery<VariantProductsResult>(variantProductsQuery, {
2 productsRef: newProducts.map((product) => product.ref),
3 catalogueRef: `MASTER:${activeRetailer}`, // TODO product catalogue ref (for example, DEFAULT or MASTER)
4 }).then((resp) => {
Language: typescript
Name: src/components/AddProductItems/AddProductItemsModal.tsx:208
Description:
[Warning: empty required content area]1 return {
2 ref: item.ref,
3 productRef: item.ref,
4 productCatalogueRef: `MASTER:${activeRetailer}`, // TODO product catalogue ref (for example, DEFAULT or MASTER)
5 quantity: item.quantity,
6 paidPrice: item.price,
Language: typescript
Name: src/forms/fields/order/СreateOrder/CreateOrder.tsx:353
Description:
[Warning: empty required content area]1 return {
2 ref: product.ref,
3 productRef: product.ref,
4 productCatalogueRef: `MASTER:${activeRetailer}`, // TODO product catalogue ref (for example, DEFAULT or MASTER)
5 quantity: product.quantity,
6 paidPrice: product.price,
Language: typescript
Name: src/forms/fields/order/СreateOrder/CreateOrder.tsx:417
Description:
[Warning: empty required content area]- Hardcoded Fulfilment Option type
1return getQuery<FulfilmentOptionResult>(createFulfilmentPlansMutation, {
2 uuid: `App-${uuid()}`,
3 type: orderType, // TODO type of FulfilmentOption entity (for example, DEFAULT or HD/CC (orderType))
4 orderType: orderType,
5 retailerId: activeRetailer,
Language: typescript
Name: src/queries/createFulfilmentPlans.tsx:88
Description:
[Warning: empty required content area]After order creation, the user will be navigated to the new order details page (by default:
`#/orders/{orderId}/{activeRetailerId}/{orderRef}`
`CreateOrder.tsx`
1window.location.hash = TemplateRegistry.render(
2 `#/orders/${resp.data.createOrder.id}/${activeRetailer}/${resp.data.createOrder.ref}`,
3);
Language: typescript
Name: src/forms/fields/order/СreateOrder/CreateOrder.tsx:446-448
Description:
[Warning: empty required content area]The full source code is presented in the section 'Related Sources'.
Manifest changes: Create Order user action definition
The button for triggering the Create Order wizard drawer can be added as a mutation user action by updating the application manifest.
1{
2 "actions": {
3 "primary": [
4 {
5 "type": "mutation",
6 "label": "i18n:fc.se.field.create.order.user.action.label",
7 "name": "createComment",
8 "filter": {
9 "type": "include",
10 "names": [
11 "text"
12 ]
13 },
14 "args": {
15 "entityId": "-1",
16 "entityType": "i18n:fc.om.orders.index.userAction.entityType.order"
17 },
18 "overrides": {
19 "text": {
20 "component": "createOrderWizard"
21 }
22 }
23 }
24 ]
25 }
26}
Language: json
Name: Create Order action definition
Description:
[Warning: empty required content area]
Setting Updates
List of settings that must be present in the system for correct work of the Create Order wizard:
- - name of catalog to provide product availability information for 'Add Item' modal.
`AGGREGATE_CATALOGUE_FOR_ADD_PRODUCT_ITEMS`
Property | Value |
Name | AGGREGATE_CATALOGUE_FOR_ADD_PRODUCT_ITEMS |
Context | ACCOUNT |
Context Id | 0 |
Value Type | STRING |
Value | Name of catalogue (for example: VC:AGGREGATE) |
- - list of Order Types with localization labels to be used on Create Order Wizard.
`ORDER_TYPES`
Property | Value |
Name | ORDER_TYPES |
Context | ACCOUNT |
Context Id | 0 |
Value Type | JSON |
Value | List of Order Types with labels (see example) |
1[
2 {
3 "label": "{{i18n 'fc.se.field.create.order.click.and.collect'}}",
4 "value": "CC"
5 },
6 {
7 "label": "{{i18n 'fc.se.field.create.order.home.delivery'}}",
8 "value": "HD"
9 }
10]
Language: javascript
Name: ORDER_TYPES value example
Description:
[Warning: empty required content area]- - list of Card Types that are available to select for Financial Transactions.
`PAYMENT.CARDTYPE`
Property | Value |
Name | PAYMENT.CARDTYPE |
Context | RETAILER |
Context Id | <retailer_id> |
Value Type | JSON |
Value | List of Card Types (see example) |
1[
2 {
3 "name": "MASTERCARD"
4 },
5 {
6 "name": "VISA"
7 },
8 {
9 "name": "GIFTCARD"
10 },
11 {
12 "name": "AMEX"
13 },
14 {
15 "name": "DISCOVER"
16 },
17 {
18 "name": "UNIONPAY"
19 },
20 {
21 "name": "JCB"
22 },
23 {
24 "name": "MAESTRO"
25 },
26 {
27 "name": "INTERAC"
28 }
29]
Language: json
Name: PAYMENT.CARDTYPE value example
Description:
[Warning: empty required content area]- - tax information to be used by default (see
`DEFAULT_TAX_TYPE`
).`src/hooks/useDefaultTariffValue.tsx`
Property | Value |
Name | DEFAULT_TAX_TYPE |
Context | RETAILER |
Context Id | <retailer_id> |
Value Type | JSON |
Value | Default tax tariff name |
1{
2 "country": "GB",
3 "group": "STANDARD",
4 "tariff": "VAT"
5}
Language: json
Name: DEFAULT_TAX_TYPE value example
Description:
[Warning: empty required content area]Additionally, a setting named as tariff name has to exist. This setting shows the amount of tax.
Property | Value |
Name | tariff name (for example: VAT) |
Context | RETAILER |
Context Id | <retailer_id> |
Value Type | STRING |
Value | Tax amount (for example, 0.2) |
- - list of currencies that are available to select for Financial Transactions.
`PAYMENT.CURRENCY`
Property | Value |
Name | PAYMENT.CURRENCY |
Context | RETAILER |
Context Id | <retailer_id> |
Value Type | JSON |
Value | List of available currencies |
1[
2 {
3 "name": "AUD"
4 },
5 {
6 "name": "NZD"
7 },
8 {
9 "name": "USD"
10 },
11 {
12 "name": "GBP"
13 },
14 {
15 "name": "EUR"
16 },
17 {
18 "name": "SGD"
19 },
20 {
21 "name": "INR"
22 },
23 {
24 "name": "MXN"
25 }
26]
Language: json
Name: PAYMENT.CURRENCY value example
Description:
[Warning: empty required content area]- - list of payment methods that are available to select for Financial Transactions.
`PAYMENT.METHOD`
Property | Value |
Name | PAYMENT.METHOD |
Context | RETAILER |
Context Id | <retailer_id> |
Value Type | JSON |
Value | List of available payment methods |
1[
2 {
3 "name": "CREDITCARD"
4 },
5 {
6 "name": "PAYPAL"
7 },
8 {
9 "name": "GIFTCARD"
10 },
11 {
12 "name": "EFT"
13 },
14 {
15 "name": "CASH"
16 },
17 {
18 "name": "AFTERPAY"
19 }
20]
Language: json
Name: PAYMENT.METHOD value example
Description:
[Warning: empty required content area]- - list of payment providers that are available to select for Financial Transactions.
`PAYMENT.PROVIDER`
Property | Value |
Name | PAYMENT.PROVIDER |
Context | RETAILER |
Context Id | <retailer_id> |
Value Type | JSON |
Value | List of available payment providers |
1[
2 {
3 "name": "BRAINTREE"
4 },
5 {
6 "name": "CYBERSOURCE"
7 },
8 {
9 "name": "PAYPAL"
10 },
11 {
12 "name": "GIVEX"
13 },
14 {
15 "name": "AFTERPAY"
16 },
17 {
18 "name": "FAT_ZEBRA"
19 },
20 {
21 "name": "BASIC_CREDIT"
22 }
23]
Language: json
Name: PAYMENT.PROVIDER value example
Description:
[Warning: empty required content area]The list of constants for related settings is described in the file
`src/constants/SettingNames.ts`
Localization Updates
List of localization entries which was used in 'Create Order Wizard' component and related settings:
1{
2 "translation": {
3 "fc.se.field.create.order.user.action.label": "Create Order",
4 "fc.se.wizard.subtitle": "Step {{activeStep}} of {{count}}",
5 "fc.se.wizard.button.previous": "Previous",
6 "fc.se.wizard.button.next": "Next",
7 "fc.se.wizard.button.skip": "Skip",
8 "fc.se.field.create.order.title": "Create Order",
9 "fc.se.field.create.order.first.step.title": "Choose product items",
10 "fc.se.field.create.order.second.step.title": "Choose customer and order type",
11 "fc.se.field.create.order.second.step.customer.label": "Customer",
12 "fc.se.field.create.order.second.step.order.type.label": "Order Type",
13 "fc.se.field.create.order.home.delivery": "Home Delivery",
14 "fc.se.field.create.order.click.and.collect": "Click and Collect",
15 "fc.se.field.create.order.second.step.customer.address.label": "Customer Address",
16 "fc.se.field.create.order.second.step.street.label": "Street",
17 "fc.se.field.create.order.second.step.city.label": "City",
18 "fc.se.field.create.order.second.step.postcode.label": "Zip",
19 "fc.se.field.create.order.second.step.state.label": "State",
20 "fc.se.field.create.order.second.step.country.label": "Country",
21 "fc.se.field.create.order.second.step.location": "Location",
22 "fc.se.field.create.order.third.step.title": "Choose delivery option",
23 "fc.se.field.create.order.fulfilment.plans.hour": "Hour",
24 "fc.se.field.create.order.fulfilment.plans.hours": "Hours",
25 "fc.se.field.create.order.fulfilment.plans.delivery.type": "Delivery Service Level",
26 "fc.se.field.create.order.fulfilment.plans.location": "Location",
27 "fc.se.field.create.order.multi.collection.items.product": "Product",
28 "fc.se.field.create.order.multi.collection.items.ref.no": "Ref no",
29 "fc.se.field.create.order.multi.collection.quantity": "Quantity",
30 "fc.se.field.create.order.multi.collection.split.btn": "Split",
31 "fc.se.field.create.order.multi.collection.address.title": "Address",
32 "fc.se.field.create.order.multi.collection.address.modal": "Address",
33 "fc.se.field.create.order.multi.collection.change.address": "Change Address",
34 "fc.se.field.create.order.multi.collection.add.address": "Add Address",
35 "fc.se.field.create.order.multi.collection.order.type.label": "Order Type",
36 "fc.se.field.create.order.multi.collection.location": "Location",
37 "fc.se.field.create.order.multi.collection.split.item.label": "Split Item",
38 "fc.se.field.create.order.multi.collection.split.done.btn": "Done",
39 "fc.se.field.create.order.multi.collection.address.form.street.label": "Street",
40 "fc.se.field.create.order.multi.collection.address.form.city.label": "City",
41 "fc.se.field.create.order.multi.collection.address.form.postcode.label": "Zip",
42 "fc.se.field.create.order.multi.collection.address.form.state.label": "State",
43 "fc.se.field.create.order.multi.collection.address.form.country.label": "Country",
44 "fc.se.field.create.order.multi.collection.address.save.btn": "Save",
45 "fc.se.field.create.order.multi.collection.address.close.btn": "Close",
46 "fc.se.field.create.order.multi.collection.default.delivery.address": "Default Delivery Address",
47 "fc.se.field.create.order.multi.collection.default.customer.address": "Default Customer Address",
48 "fc.se.field.create.order.third.step.basket": "Basket",
49 "fc.se.field.create.order.third.step.item.collection": "Item pickup",
50 "fc.se.field.create.order.fifth.step.financial.transaction.title": "Financial Transactions",
51 "fc.se.field.create.order.fifth.step.financial.transaction.add.button": "Add Financial Transaction",
52 "fc.se.field.create.order.fifth.step.financial.transaction.remove.button": "Remove Financial Transaction",
53 "fc.se.field.create.order.fifth.step.financial.transaction.amount": "Amount",
54 "fc.se.field.create.order.fifth.step.financial.transaction.currency": "Currency",
55 "fc.se.field.create.order.fifth.step.financial.transaction.payment.method": "Payment Method",
56 "fc.se.field.create.order.fifth.step.financial.transaction.payment.provider": "Payment Provider",
57 "fc.se.field.create.order.fifth.step.financial.transaction.ref": "Transaction Ref",
58 "fc.se.field.create.order.fifth.step.financial.transaction.card.type": "Card Type",
59 "fc.se.field.create.order.summary.title": "Summary",
60 "fc.se.field.create.order.summary.product.list.head.image": "Image",
61 "fc.se.field.create.order.summary.product.list.head.product": "Product",
62 "fc.se.field.create.order.summary.product.list.head.price": "Price",
63 "fc.se.field.create.order.summary.product.list.head.quantity": "Quantity",
64 "fc.se.field.create.order.summary.order.type": "Order Type",
65 "fc.se.field.create.order.summary.delivery.type": "Delivery Service Level",
66 "fc.se.field.create.order.summary.country": "Country",
67 "fc.se.field.create.order.summary.city": "City",
68 "fc.se.field.create.order.summary.state": "State",
69 "fc.se.field.create.order.summary.street": "Street",
70 "fc.se.field.create.order.summary.postcode": "Zip",
71 "fc.se.field.create.order.summary.pickup.location": "Pickup Location",
72 "fc.se.field.create.order.summary.taxes": "Tax",
73 "fc.se.field.create.order.summary.subtotal": "Subtotal",
74 "fc.se.field.create.order.summary.total.price": "Order Total",
75 "fc.se.field.create.order.summary.delivery.details": "Delivery Details",
76 "fc.se.field.create.order.summary.order.items": "Order Items",
77 "fc.se.field.create.order.summary.item.image": "Item Image",
78 "fc.se.field.create.order.summary.item.name": "Item Name",
79 "fc.se.field.create.order.summary.quantity": "Quantity",
80 "fc.se.field.create.order.summary.price": "Price",
81 "fc.se.field.create.order.summary.total.item.price": "Total Item Price",
82 "fc.se.field.create.order.summary.order.item.type": "Order Item Type",
83 "fc.se.field.create.order.summary.address.label": "Address",
84 "fc.se.field.create.order.bottom.required.label": "Required",
85 "fc.se.field.create.order.not.complete.steps": "Please complete all steps",
86 "fc.se.field.create.order.success.created.order": "You successfully created order",
87 "fc.se.field.create.order.successfully.created.order.message": "Order {{ref}} for customer {{name}} was successfully created",
88 "fc.se.add.product.items.item.added": "Item added",
89 "fc.se.add.product.items.item.added.to.order": "has been added to order",
90 "fc.se.add.product.items.error.label": "Error",
91 "fc.se.add.product.items.error.empty.setting": "There is no setting {{settingName}}",
92 "fc.se.add.product.items.error.not.aggregate.setting": "Catalog in {{settingForDefaultCatalogue}} setting should be aggregate",
93 "fc.se.add.product.items.product": "Product",
94 "fc.se.add.product.items.ref.no": "Ref no",
95 "fc.se.add.product.items.quantity": "Quantity",
96 "fc.se.add.product.items.unit.price": "Unit price",
97 "fc.se.add.product.items.was": "was",
98 "fc.se.add.product.items.taxes": "Tax",
99 "fc.se.add.product.items.subtotal": "Subtotal",
100 "fc.se.add.product.items.total.price": "Order Total",
101 "fc.se.add.product.items.add.modal.search.product.ref": "Product Ref",
102 "fc.se.add.product.items.add.modal.title": "Select Products",
103 "fc.se.add.product.items.add.modal.button.done": "Done",
104 "fc.se.add.product.items.add.modal.table.head.image": "Image",
105 "fc.se.add.product.items.add.modal.table.head.product": "Product",
106 "fc.se.add.product.items.add.modal.table.head.attributes": "Attributes",
107 "fc.se.add.product.items.add.modal.table.show.attributes.title": "Attributes",
108 "fc.se.add.product.items.add.modal.table.head.quantity": "Quantity",
109 "fc.se.add.product.items.add.modal.table.head.add": "Add",
110 "fc.se.add.product.items.add.modal.showing.rows": "Showing {{start}} of {{end}}",
111 "fc.se.add.product.items.add.modal.table.no.available": "No available Products",
112 "fc.se.add.product.items.add.item.button": "Add item",
113 "fc.se.add.product.items.product.list.head.image": "Image",
114 "fc.se.add.product.items.product.list.head.product": "Product",
115 "fc.se.add.product.items.product.list.head.price": "Net Price",
116 "fc.se.add.product.items.product.list.head.description": "Description",
117 "fc.se.add.product.items.product.list.head.remove": "Remove",
118 "fc.se.add.product.items.product.list.head.quantity": "Quantity",
119 "fc.se.add.product.items.product.list.attributes.modal.title": "Product Attributes",
120 "fc.se.add.product.items.product.list.count.ofMax": "of {{max}}"
121 }
122}
123
Language: json
Name: Localization Entries
Description:
[Warning: empty required content area]
Result
'Create Order' action is placed on 'Orders' page.
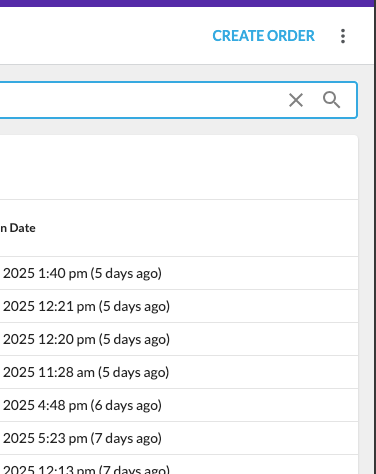
Create Order Wizard, Step 1 - select Order Items.
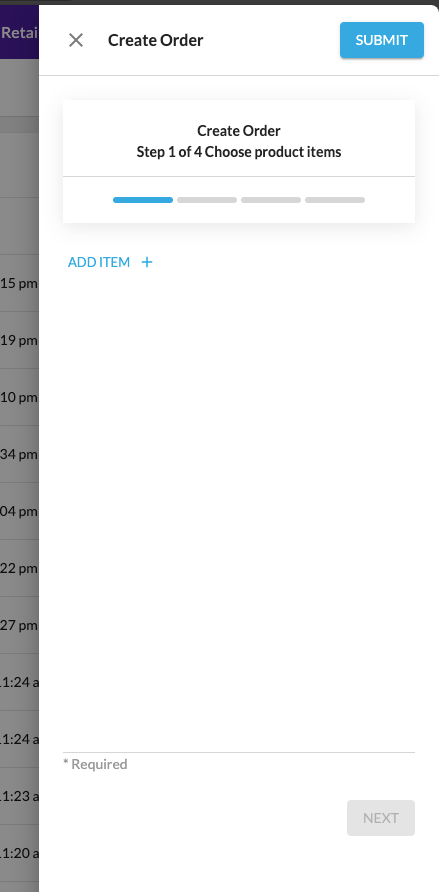
Create Order Wizard, Step 1 - modal dialog to select Order Items.
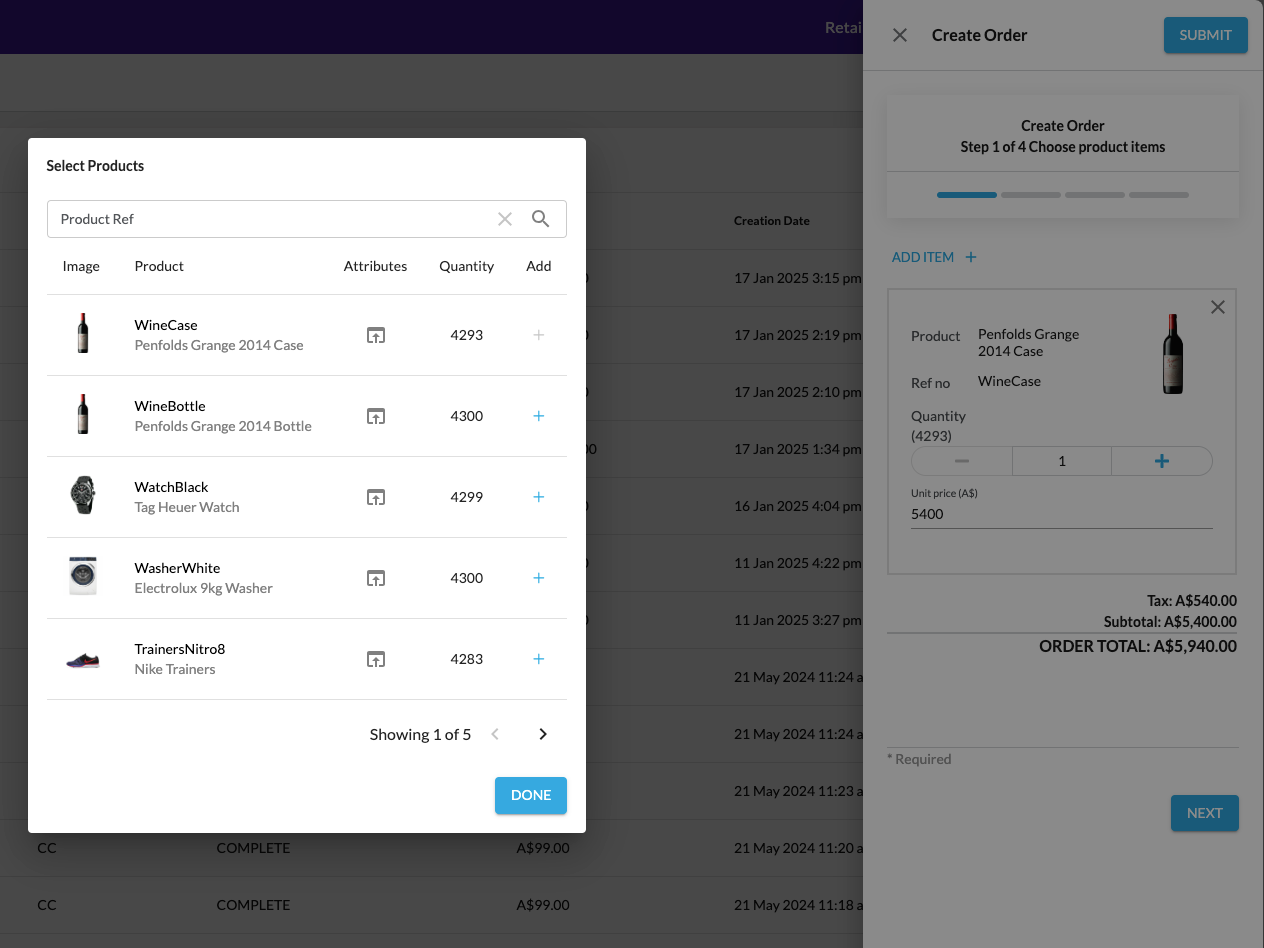
Create Order Wizard, Step 2 - select Order Type and Customer/Delivery information.
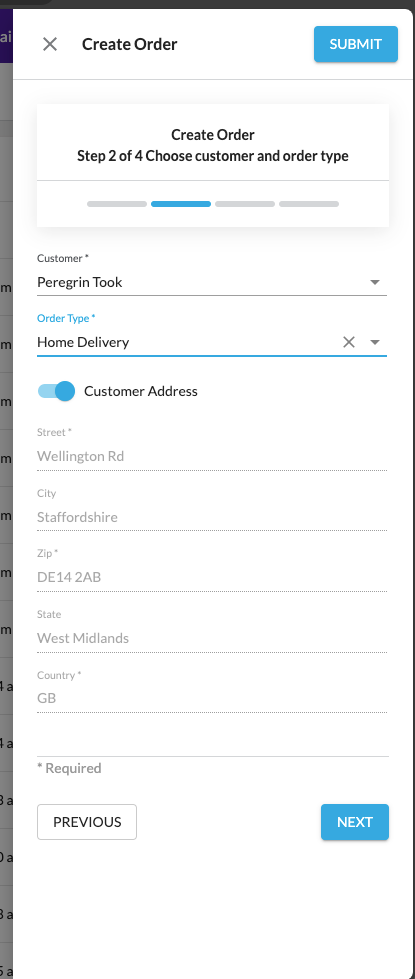
Create Order Wizard, Step 3 - select Delivery Type/Carrier.
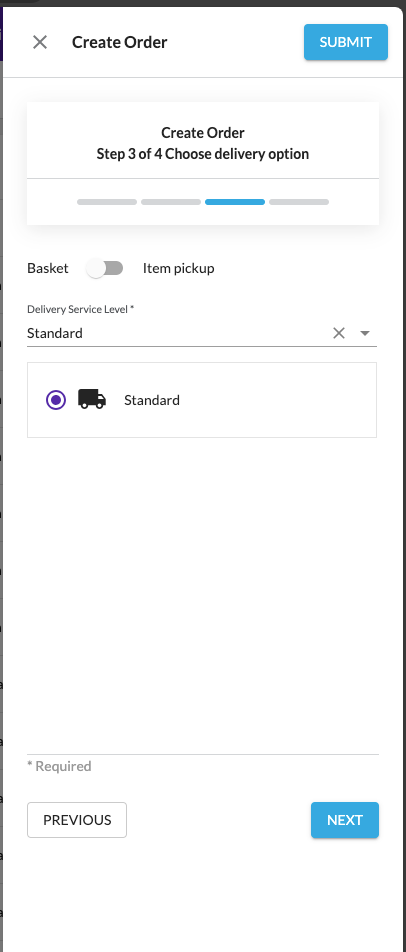
Create Order Wizard, Step 3 - configure Delivery for Mixed Order.
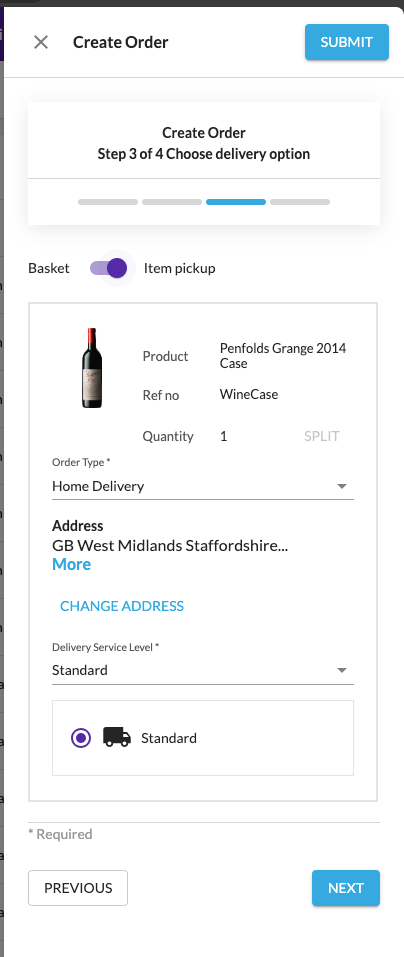
Create Order Wizard, Step 4 - add information about Financial Transactions.
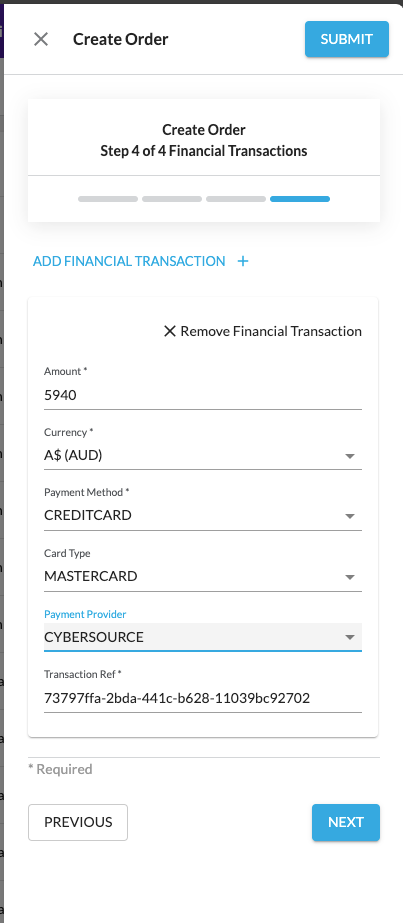
Create Order Wizard, Final Step - Summary.
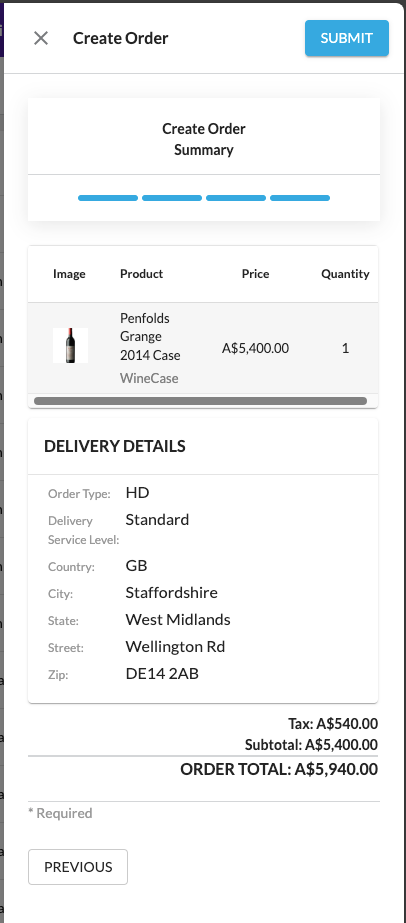