OMS Web app: ability to search order by ID
Author:
Randy Chan
Changed on:
30 Apr 2025
Key Points
- This is the use case where users can look up OMS orders using order ID.
- Typically, the graphQL query orderById will be used to look up an order by ID. However, they can only look up one order at a time and with no wildcard search.
- This article provides an alternative approach that allows users to search for orders by ID or even with a wildcard.
- The approach is to utilise the recent release of the ref2 field in order entity.
Steps
Steps to achieve the outcome
- Create a custom rule (by using rules SDK)
`CopyOrderIdToOrderRef2`
- Add the rule to the order workflows
`CopyOrderIdToOrderRef2`
- Extend the Order list screen manifest to include fc.filterPanel with ref2
- Test the outcome
Create a custom rule CopyOrderIdToOrderRef2 (by using rules SDK)
Open the rule SDK and create a new java rule: CopyOrderIdToOrderRef2
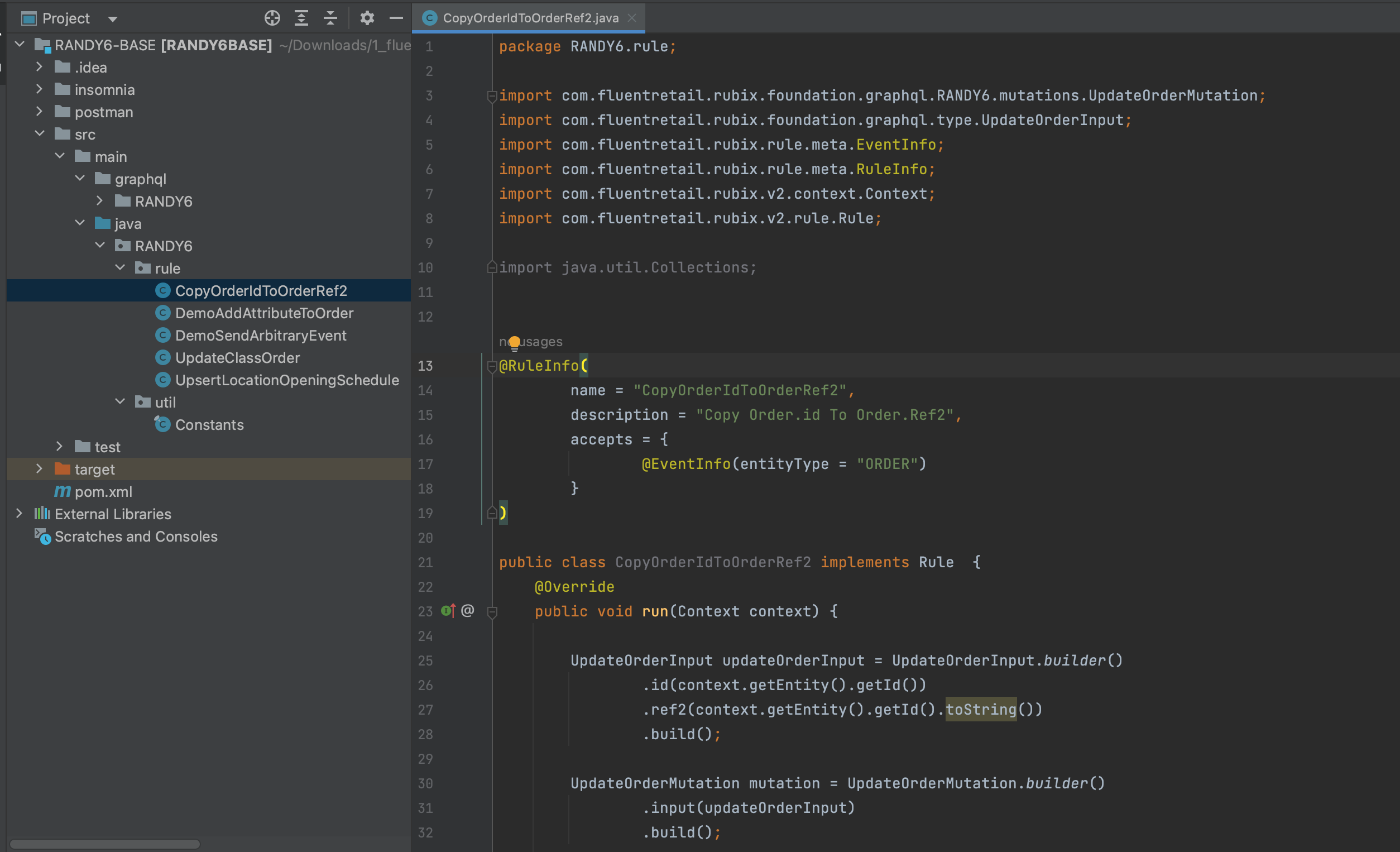
1package RANDY6.rule;
2
3import com.fluentretail.rubix.foundation.graphql.RANDY6.mutations.UpdateOrderMutation;
4import com.fluentretail.rubix.foundation.graphql.type.UpdateOrderInput;
5import com.fluentretail.rubix.rule.meta.EventInfo;
6import com.fluentretail.rubix.rule.meta.RuleInfo;
7import com.fluentretail.rubix.v2.context.Context;
8import com.fluentretail.rubix.v2.rule.Rule;
9
10import java.util.Collections;
11
12
13@RuleInfo(
14 name = "CopyOrderIdToOrderRef2",
15 description = "Copy Order.id To Order.Ref2",
16 accepts = {
17 @EventInfo(entityType = "ORDER")
18 }
19)
20
21public class CopyOrderIdToOrderRef2 implements Rule {
22 @Override
23 public void run(Context context) {
24
25 UpdateOrderInput updateOrderInput = UpdateOrderInput.builder()
26 .id(context.getEntity().getId())
27 .ref2(context.getEntity().getId().toString())
28 .build();
29
30 UpdateOrderMutation mutation = UpdateOrderMutation.builder()
31 .input(updateOrderInput)
32 .build();
33
34 context.action().mutation(mutation);
35 }
36}
37
Language: java
Name: CopyOrderIdToOrderRef2 java
Description:
CopyOrderIdToOrderRef2 java
Then, compile to jar and install the plugin onto your account.
Use GET: {{fluentApiHost}}/orchestration/rest/v1/plugin to check the available new rule.
Add the rule CopyOrderIdToOrderRef2 to the order workflows
Once CopyOrderIdToOrderRef2 is deployed, now you can add a new rule into the CREATE ruleset in the Order workflows (HD and CC):
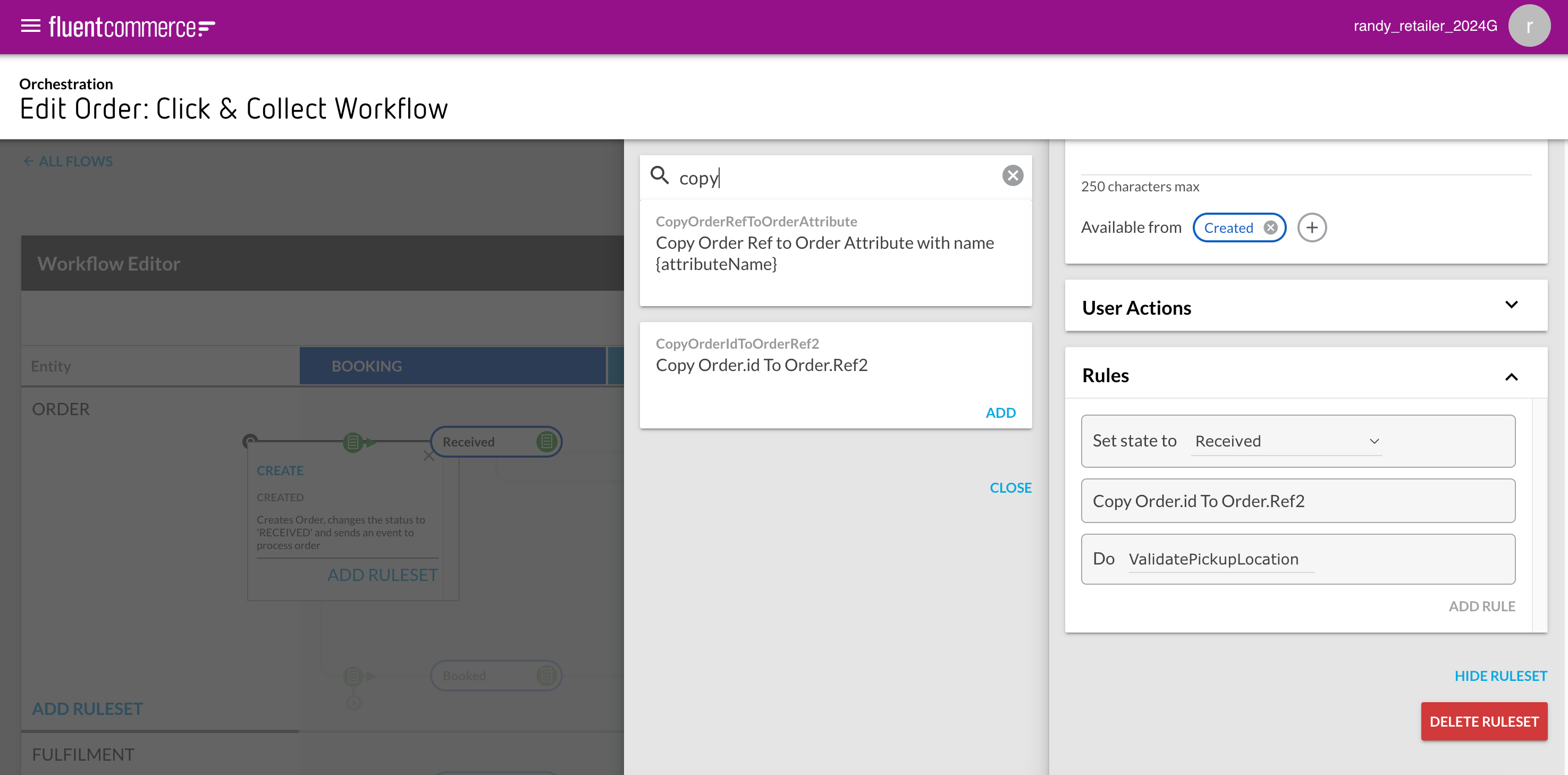
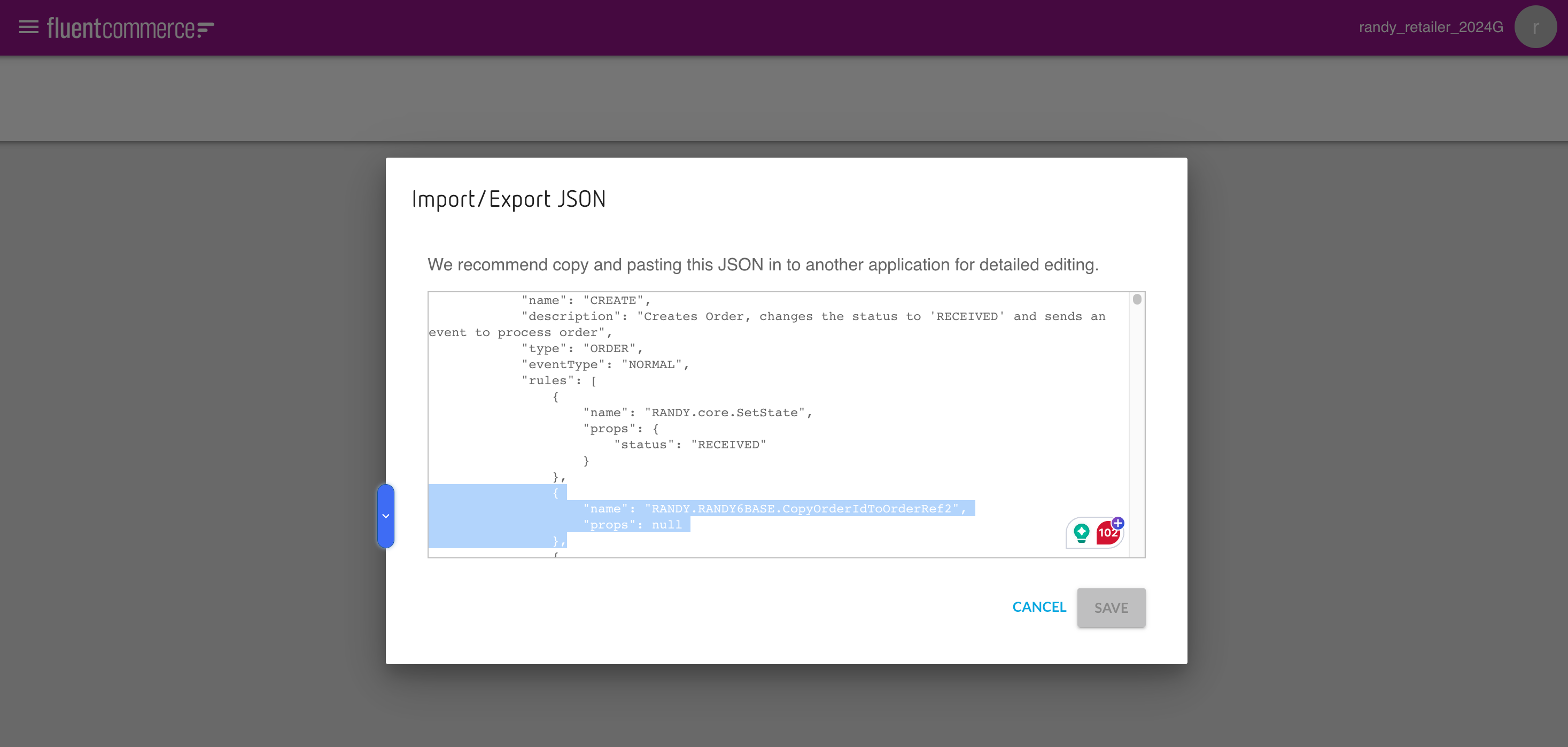
1{
2 "name": "{{AccountID}}.{{packageName}}.CopyOrderIdToOrderRef2",
3 "props": null
4},
Language: json
Name: CopyOrderIdToOrderRef2 rule in ruleset
Description:
[Warning: empty required content area]Save the change.
Extend the Order list screen manifest to include fc.filterPanel with ref2
This will be done in the settings: fc.mystique.manifest.oms.fragment.ordermanagement
First, add fc.filterPanel above the fc.list:
1"descendants": [
2{
3 "component": "fc.filterPanel",
4 "props": {
5 "filtersSource": "orders",
6 "allowReadWriteUrlParams": true,
7 "additionalFields": [],
8 "overrides": {
9 "ref": {
10 "component": "fc.field.multistring",
11 "label": "Order ref",
12 "exactSearch": false,
13 "visibleItemsThreshold": 3
14 },
15 "ref2": {
16 "component": "fc.field.multistring",
17 "label": "Order ref2",
18 "exactSearch": false,
19 "visibleItemsThreshold": 3
20 }
21 },
22 "exclude": [
23 "productRef",
24 "createdon",
25 "updatedOn",
26 "quantity",
27 "paidPrice",
28 "currency",
29 "retailerId",
30 "price",
31 "taxPrice",
32 "taxType",
33 "totalPrice",
34 "totalTaxPrice",
35 "type",
36 "status",
37 "tag1",
38 "tag2",
39 "tag3"
40 ]
41 }
42},
43{
44 "component": "fc.list",
45 "props": {
46 "defaultPageSize": 100,
47 "dataSource": "orders",
48...
Language: json
Name: fc.filterPanel
Description:
[Warning: empty required content area]Then, for testing purposes, add the following order id and ref2 to the fc.list -> attributes section:
1{
2 "label": "order id",
3 "template": "{{node.id}}"
4},
5{
6 "label": "ref2",
7 "template": "{{node.ref2}}"
8},
Language: json
Name: fc.list attributes
Description:
[Warning: empty required content area]Save the change
Test the outcome
Create a few new orders, and the new workflow will populate ref2. Then go to Order List page (refresh the browser to get the latest manifest):
search order by ID using ref2 field
search order by ID using ref2 field