External Network Notification
Author:
Fluent Commerce
Changed on:
9 Oct 2023
Overview
In this sample project, we will go through some basic features of the SDK by creating a connector that can receive notifications from Fluent System, which is processed and sent to any external system like Email, Slack, Datadog, etc.
These are the topics covered by this guide:
- Create a new Handler for receiving Notification from Fluent System
- Send Notifications to an external network
- Schedule Job Handler for Monitoring failed events
External Network Notification
Author:
Fluent Commerce
Changed on:
9 Oct 2023
Key Points
- Prerequisites
- Script Execution
- Create a new Handler for receiving notifications from Fluent Platform
- Schedule Job Handler to Monitoring failed events
Steps
Prerequisites
- localstack container is running
- You have access to a Fluent account
There is a script
`localstack-setup.sh`
It is best to do the following:
First, run this command to open a session with the localstack container
1docker exec -it localstack /bin/bash
Language: java
Name: Command
Description:
[Warning: empty required content area]Then use the commands below to create the secrets, but ensure to update the variables: $ACCOUNT, $RETAILER, $USERNAME, $PASSWORD and $REGION. Regions values are: sydney, dublin, singapore or north_america.
- API key/secret for communicating with external network
When communicating with an external network/platform from its client API interface, it always requires some kind of key or token used for access.
A script
`localstack-setup.sh`
Script Execution:
First, run this command to open a session with the localstack container
docker exec -it localstack /bin/bash Then use the commands below to create the secrets. Make sure to update the variables: $ACCOUNT, $EMAIL_SECRET_KEY, $EMAIL_SENDGRID_API_KEY. Please check the localstack-setup.sh script for more details.
For Email:
1awslocal secretsmanager create-secret --name fc/connect/external-network-notification/$ACCOUNT/$EMAIL_SECRET_KEY --secret-string "{\"apiKey\" : $EMAIL_SENDGRID_API_KEY }";
Language: java
Name: Command
Description:
[Warning: empty required content area]For Slack:
1awslocal secretsmanager create-secret --name fc/connect/external-network-notification/$ACCOUNT/$SLACK_SECRET_KEY --secret-string "{\"webHookUrl\" : \"$SLACK_WEBHOOK_URL\" }"
Language: java
Name: Command
Description:
[Warning: empty required content area]
Create a new Handler for receiving notifications from Fluent Platform:
SDK Default Handler:
A default implementation of [NotificationHandler] i.e. [DefaultNotificationHandler] is provided by the SDK
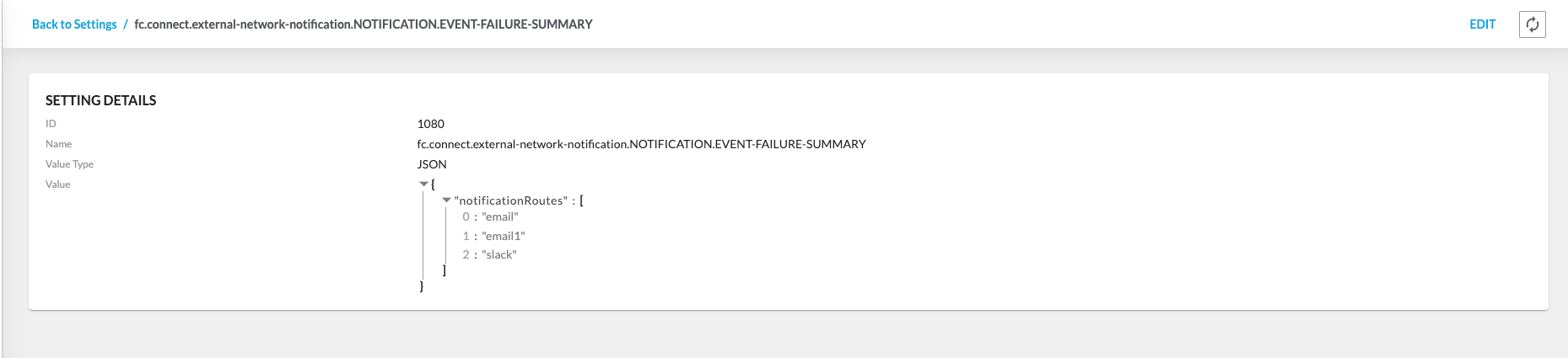
Note that this handler will read the setting from Fluent OMS with the key:
`fc.connect.external-network-notification.<connectorName>.<type>`
The setting has the following format :
1{
2 "notificationRoutes": [
3 "email",
4 "slack"
5 ]
6}
Language: json
Name: Example
Description:
[Warning: empty required content area]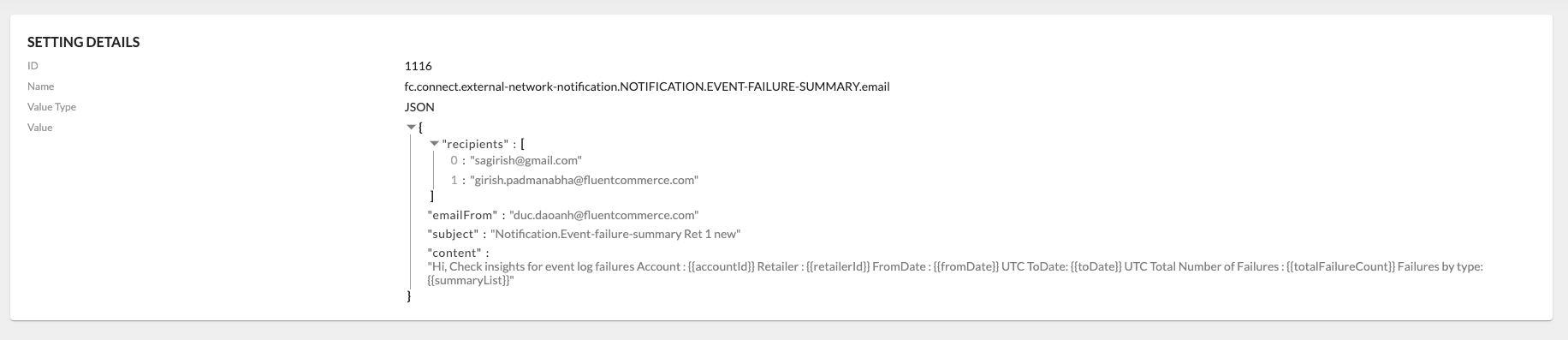
Note that this handler will read the setting from Fluent OMS with the key:
`fc.connect.external-network-notification.<connectorName>.<type>.<notificationType>`
The setting has the following format :
1{
2 "recipients": [
3 "to_0@example.com",
4 "to_1@example.com"
5 ],
6 "emailFrom": "from@example.com",
7 "subject": "Notification.Event-failure-summary",
8 "content": "Hi,\nCheck insights for event log failures\n\nAccount : {{accountId}} \nRetailer : {{retailerId}}\nFromDate : {{fromDate}} UTC\nToDate: {{toDate}} UTC\n\nTotal Number of Failures : {{totalFailureCount}} \nFailures by type: {{summaryList}}"
9}
Language: java
Name: Example
Description:
[Warning: empty required content area]Custom Email Handler:
Any new Notification handler must implement the interface
`NotificationHandler`
Take EmailNotificationHandler as an example:
1@Slf4j
2@Component
3@HandlerInfo(name = "EmailNotificationHandler", route = "email")
4public class EmailNotificationHandler implements NotificationHandler {
5
6
7 // For example only. Don't put it here. This can be placed inside EmailNotificationData
8 private static final String SECRET_NAME = "send-grid";
9 private final EmailTemplateProcessor templateProcessor;
10
11 @Autowired
12 public EmailNotificationHandler(final EmailTemplateProcessor templateProcessor) {
13 this.templateProcessor = templateProcessor;
14 }
15
16 /**
17 * Handle notification to send email with SendGrid. This method execution can be refactored into another
18 * service to dynamically handle mail sending process and/or any other Mail client dependency.
19 *
20 * @param context context includes the message to be processed along with some common used SDK services.
21 */
22 @Override
23 public void processNotification(@NotNull final NotificationHandlerContext context) {
24 // Configuration from OMS setting .
25 final var data = context.getNotificationConfiguration(EmailNotificationData.class, EmailNotificationData.empty());
26 // Payload that will contain the referencing values that later applied to raw content of the mail template.
27 final var referencingPayload = context.getMessage().getPayload();
28 final var rawContent = data.content();
29 var updatedEmailContent = templateProcessor.processEmailContent(rawContent, referencingPayload);
30 Mail mail = toMail(data, updatedEmailContent);
31 sendEmail(mail, prepareSendGrid(context));
32 }
33
34
35 // Removed for brevity
36}
Language: java
Name: Example
Description:
[Warning: empty required content area]Handling Notification
- The method is the entry point for the handler. It will be called by the SDK when a new Notification Event is published.
`processNotification`
- The parameter contains the message to be processed along with some common used SDK services:
`context`
- : POJO object that contains the FluentOMS Settings for sending the email :
`EmailNotificationData`
,`sendFrom`
,`sendTo`
,`subject`
....`rawContent`
- : A Mapping for the referencing value for the Email Raw Content. > For example: > > You have a raw content of the mail defined inside
`referencingPayload`
like: > >`FluentOMS Settings`
> > The {{customerName}} will be replaced by the value of`Dear {{customerName}},....`
by the actual value specified in the message data.`customerName`
ProcessingEmail Content:
- The conversion from message template to final email content is very basic in this example and the intent is to simply demonstrate the feature - NotificationTemplateProcessor. Please consider proper templating libraries when using it in a production environment.
Sending Email:
For the current example, we are using SendGrid as the email client.
1private static SendGrid prepareSendGrid(@NotNull final NotificationHandlerContext context) {
2 String accountId = context.getAccountReference().accountId();
3 final Credential credential = context.getCredentialService().getCredentials(accountId, Collections.singleton(SECRET_NAME));
4 Optional<SendGridData> secret = credential.getSecret(SECRET_NAME, SendGridData.class);
5 if (secret.isEmpty()) {
6 throw new SecretNotFoundException("SendGrid cannot be initialized");
7 }
8 return new SendGrid(secret.get().apiKey());
9}
Language: java
Name: Example
Description:
[Warning: empty required content area]- This class scope method is used to initialize the
`prepareSendGrid`
client. The`SendGrid`
is a POJO object that contains the apiKey for the`SendGridData`
client.`SendGrid`
- The SendGridData is retrieved from the Credential service. The Credential service is used to retrieve the secret from the Secrets Manager of the localstack.
Slack Notification Handler:
We are going to follow the same approach as the Email Handler.
This sample implementation is used for both email and slack: DefinedTemplateNotificationHandler.
The only difference is how we are going to set up mandatory configuration as a Slack client to send notification.
For detail steps, please refer to this link Sending Message Using Slack Web hook.
Schedule Job Handler to Monitoring failed events
Trigger a Job:
1curl -X PUT http://localhost:8080/api/v1/fluent-connect/scheduler/add/<ACCOUNT>/<RETAILER>/event-failure-summary-monitor
Language: java
Name: Command
Description:
[Warning: empty required content area]Example of the CURL with the replaced values:
1curl -X PUT http://localhost:8080/api/v1/fluent-connect/scheduler/add/cnctdev/34/event-failure-summary-monitor
Language: java
Name: Example
Description:
[Warning: empty required content area]