Unit Testing Custom Components
Authors:
Cameron Johns, Cille Schliebitz, Anita Gu
Changed on:
3 Feb 2025
Overview
This lesson covers the process of unit testing custom components using the tools provided within the Component SDK: Jest and React's test renderer. We'll walk through the steps of creating mock objects for dependencies (authentication, data, attributes), arranging the test environment, acting by rendering the component with the mocks, and asserting the output using snapshot testing. This process ensures the reliability and correctness of your custom components.
Key points
- Testing Framework: The SDK includes Jest and React's test renderer for unit testing.
- Mock Objects: Mock objects are created for external dependencies like authentication (), data from the page query, and dynamic attributes from the manifest.
`useAuth`
- Arrange, Act, Assert: The testing process follows the standard Arrange, Act, Assert pattern.
- Arrange: Mocks are set up and configured.
- Act: The component is rendered with the mock data.
- Assert: The rendered output is compared against a snapshot.
- Snapshot Testing: Jest's snapshot feature is used to compare the component's output to a previously saved snapshot, ensuring consistency.
- Testable Components: Unit tests help ensure the reliability and maintainability of custom components.
The SDK comes pre-packaged with the Jest mocking framework and React's test renderer.
Unit testing components involve mocking out external dependencies and asserting behaviour of the component. You will use Jest's snapshot feature, which allows the test framework to compare the output of the component against the snapshot.
The steps are as follows:
- Create static mock objects:
- - mock the response for the
`useAuthResponse`
hook`useAuth`
- - mock the data result from the page query
`data`
- - mock the manifest configured dynamic attributes
`attributes`
- Arrange: The Arrange step sets up the mocks. In this case, we simply need to tell the useAuth hook to return our previously prepared mock auth response object
- Act: The Act step creates an instance of the TrainingMap component, taking in the mocked objects for page query response data and manifest configured dynamic attributes
- Assert: The Assert step compares the rendered output of the component to the previously snapshotted output
Here are the mock objects:
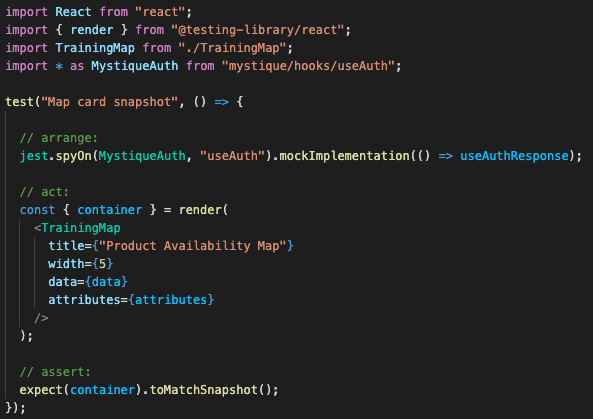
Auth Response mock:
1const useAuthResponse: MystiqueAuth.MystiqueAuth = {
2 loggedIn: true,
3 logout: () => {},
4 user: {
5 username: "F_syd@fcfashion.com.au",
6 timezone: "Australia/Sydney",
7 language: {
8 label: "English",
9 value: "en",
10 },
11 roles: [
12 {
13 role: {
14 name: "DASHBOARD_VIEW",
15 },
16 contexts: [
17 {
18 contextType: "location",
19 contextId: "4",
20 },
21 ],
22 },
23 {
24 role: {
25 name: "STORE_ASSISTANT",
26 },
27 contexts: [
28 {
29 contextType: "retailer",
30 contextId: "1",
31 },
32 {
33 contextType: "location",
34 contextId: "4",
35 },
36 ],
37 },
38 ],
39 },
40 context: {
41 level: "location",
42 current: {
43 contextType: "location",
44 contextId: "4",
45 label: "FC Fashion Sydney (F_SYD)",
46 details: {
47 ref: "F_SYD",
48 primaryAddress: {
49 latitude: -33.8845977,
50 longitude: 151.2075861,
51 },
52 },
53 },
54 available: [
55 {
56 contextType: "location",
57 contextId: "4",
58 label: "FC Fashion Sydney (F_SYD)",
59 details: {
60 ref: "F_SYD",
61 primaryAddress: {
62 latitude: -33.8845977,
63 longitude: 151.2075861,
64 },
65 },
66 },
67 ],
68 set: () => {},
69 roleCheck: () => ({ hasRole: true }),
70 },
71 };
Language: plain_text
Name: Auth Response Mock
Description:
Mocking the response for the
`useAuth`
Data mock:
1const data = {
2 edges: [
3 {
4 node: {
5 location: {
6 name: "FC Fashion Westfield Bondi Junction",
7 primaryAddress: {
8 longitude: 151.24997359266263,
9 latitude: -33.89138243611695,
10 },
11 },
12 virtualPositions: [
13 {
14 quantity: 56,
15 },
16 ],
17 },
18 },
19 {
20 node: {
21 location: {
22 name: "FC Fashion North Sydney",
23 primaryAddress: {
24 longitude: 151.2054,
25 latitude: -33.8396,
26 },
27 },
28 virtualPositions: [
29 {
30 quantity: 100,
31 },
32 ],
33 },
34 },
35 ],
36 };
Language: plain_text
Name: Data mock
Description:
Mock data for the result from the page query
Attributes mock:
1const attributes = [
2 {
3 label: "Ref",
4 value: "{{node.location.ref}}",
5 },
6 {
7 label: "Quantity",
8 value: "{{node.virtualPositions.0.quantity}}",
9 },
10 ];
Language: plain_text
Name: Attributes Mock
Description:
Mocking the manifest configured dynamic attributes
Want to learn more?
To read more about unit testing components, go to Component SDK - Geting Started > Unit Testing